Starting with a Node.js webserver

Marco Franssen /
5 min read • 1002 words
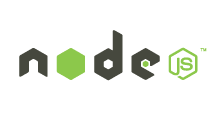
UPDATE: updated the console output to latest Node.js version and updated the express.js example to latest version.
Before starting to explain how you start your first Node.js project for building a simple web server I will first explain you what Node.js is. To do so I just include a quote of the Node.js themself, because I don't like to reinvent the wheel.
Node.js is a platform built on Chrome's JavaScript runtime for easily building fast, scalable network applications. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
The next thing you should know is about the Node.js Package Manager (NPM). Using NPM you can benefit from the thousands of open source packages so you won't have to build everything yourself.
I suppose you already have installed Node.js. Let's start with a simple Hello World in Node.js by creating a file called hello-world.js and execute our script to show the result.
console.log("Hello World");
We can execute the script using following command. (On Windows open the Node.js command prompt) Navigate to your folder containing the just created hello-world.js file and execute the script using node.
Your environment has been set up for using Node.js 4.1.1 (x64) and npm.
Press any key to continue . . .
C:\Users\Marco> cd MyHelloWorldCodeFolder
C:\Users\Marco\MyHelloWorldCodeFolder> node hello-world.js
Hello World
Ok so we have just seen our very first Node.js application running. Before we start doing some more advanced stuff I first would like to install a package which will become very handy during development of our server, called nodemon
. Nodemon will monitor our working directory and automatically restart our Node.js server when we make changes in our code. Because we want to reuse this package for all our future nodejs projects we will install this package globally using the -g
command line parameter.
Your environment has been set up for using Node.js 4.1.1 (x64) and npm.
Press any key to continue . . .
C:\Users\Marco> npm install nodemon -g
Now we can use nodemon
to execute our scripts. Lets test it by starting our script using nodemon and then make a change to our script.
Your environment has been set up for using Node.js 4.1.1 (x64) and npm.
Press any key to continue . . .
C:\Users\Marco> cd MyHelloWorldCodeFolder
C:\Users\Marco\MyHelloWorldCodeFolder> nodemon hello-world.js
23 Nov 18:49:46 - [nodemon] v0.7.10
23 Nov 18:49:46 - [nodemon] to restart at any time, enter `rs`
23 Nov 18:49:46 - [nodemon] watching: C:\Users\Marco\MyHelloWorldCodeFolder
23 Nov 18:49:46 - [nodemon] starting `node hello-world.js`
Hello World
23 Nov 18:49:46 - [nodemon] clean exit - waiting for changes before restart
Now start another console so we can install our first package. We will install the colors
package so we are able to give our console output some nice colors.
Your environment has been set up for using Node.js 4.1.1 (x64) and npm. Press any key to continue . . .
C:\Users\Marco> cd MyHelloWorldCodeFolder
C:\Users\Marco\MyHelloWorldCodeFolder> npm install colors
Then change our hello-world.js
script to the following.
var colors = require("colors");
console.log("Hello ".red + "Node.js".green);
As you can see we require the colors
package in our script so we can use it. When looking at your console you will also notice nodemon
automatically restarted your script.
23 Nov 18:50:05 - [nodemon] restarting due to changes...
23 Nov 18:50:05 - [nodemon]
C:\Users\Marco\MyHelloWorldCodeFolder\hello-world.js
23 Nov 18:50:05 - [nodemon] starting `node hello-world.js`
<span style="color: #ff0000">Hello</span> <span style="color: #008000;">Node.js</span>
23 Nov 18:50:06 - [nodemon] clean exit - waiting for changes before restart
So now we have set our development environment and we can easily develop and test our script. Since I don't like to reinvent the wheel we will use express.js
to develop our webserver. First we need to install express.js
using NPM
.
Your environment has been set up for using Node.js 4.1.1 (x64) and npm. Press any key to continue . . .
C:\Users\Marco> cd MyHelloWorldCodeFolder
C:\Users\Marco\MyHelloWorldCodeFolder> npm install express
Now we are able to use the express script in our hello-world.js
script. Lets make some changes and see nodemon automatically restarting our script and launching our webserver.
var express = require("express"),
path = require("path"),
app = express();
app.set("port", 3000);
app.use(express.static(path.join(__dirname, "/public")));
app.listen(app.get("port"), function () {
console.log("Express server listening on port %s.", app.get("port"));
});
23 Nov 19:13:01 - [nodemon] restarting due to changes...
23 Nov 19:13:01 - [nodemon] C:\Users\Marco\MyHelloWorldCodeFolders\hello-world.js
23 Nov 19:13:01 - [nodemon] starting `node hello-world.js`
Express server listening on port 3000.
Now our web server is listening on http://localhost:3000. We have configured the directory public to serve our static content. So lets add an html page to show an awesome "Hello Node.js express webserver" message. First create a folder public
and then create a file called index.html
in this folder.
<html>
<head>
<title>Hello Node.js express webserver</title>
</head>
<body>
<h1>Hello Node.js express webserver</h1>
<p>
I have just created my first node.js webserver, serving static content.
</p>
</body>
</html>
Now open your web browser again and navigate to http://localhost:3000.
In this article you have seen how to create a simple http server to serve static content. Ofcourse there are better solutions to serve static content, but imagine yourself writing some more advanced web applications in Node.js very soon. These are only the first steps to get you up and running with Node.js. Feel free to add client side Javascript, stylesheets and images to the public
folder to make your webpage some more fancy.
I hope this small guide was useful for you. Enjoy writing your own Node.js applications and share this article with your friends. Thanks for reading.