Setting up Docker development environment on Windows/Mac

Marco Franssen /
8 min read • 1502 words
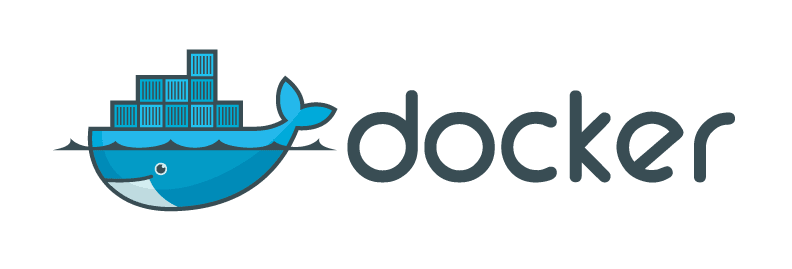
In this post I want to cover how you can setup a Docker development environment on Windows/Mac. As you might already know Docker requires a Linux kernel to run. Therefore we will always need a VM to run the actual Docker environment when you are on Windows or Mac OS. Hereby a few quotes from the Docker webpage to remember you what Docker is all about, or to give you a quick idea.
Docker provides a common framework for developer and IT teams to collaborate on applications. With a clear separation of concerns and robust tooling, organizations are able to innovate faster with greater control.
-
AGILITY
Developers have the freedom to define environments, and the ability to create and deploy apps faster and easier. IT ops have the flexibility to quickly respond to change.
-
CONTROL
Developers own all the code from infrastructure to app. IT ops have the manageability to standardize, secure, and scale the operating environment.*
-
PORTABILITY
Docker gives you choice without complexity, from a laptop to a team, to private infrastructure and public cloud providers.
Ok enough chatting, for the impatient ones continue to TL;DR. For all the others lets continue reading.
Put the whale to the water
Lets have a look how we can get an environment running on Windows or Mac. In this tutorial I'm going to use Chocolatey. Chocolatey is a package manager for Windows. It allows you to install the software you require from PowerShell. This makes it really powerful to script all the software you require on your computer by putting it in a PowerShell script. When unfamiliar with Chocolatey I recommend you to have a look at the Chocolatey webpage and install the tool as described on their page.
For the readers using a Mac, I will mention when you have to slightly differ your approach. In all the other cases you can run the same commands. For Mac I will be using a similar tool as Chocolatey, called Homebrew. When unfamiliar with this tool I assume you know how to install the packages manually or just have a look at Homebrew to figure out how to set it up on your Mac.
From this point I assume you have Chocolatey/Homebrew installed. In case you don't I'll also assume that you know how to install the required tools manually by downloading the correct installers. In order to get a VM running for our Docker environment we are going to use docker-machine to setup a docker-machine on VirtualBox. So lets start by installing Virtualbox, docker-machine and Docker using Chocolatey. In case you have any of these tools already installed you can skip this step.
Install tools
cinst -y virtualbox
cinst -y docker-machine
cinst -y docker
brew install docker-machine
brew install docker
docker-machine
Now we have installed docker-machine we are able to create a new environment. In case you already had docker-machine installed we can first have a look on what machines we have available using the following command.
$ docker-machine ls
NAME ACTIVE DRIVER STATE URL SWARM DOCKER ERRORS
default * virtualbox Running tcp://192.168.99.100:2376 v1.11.2
In my case I already have the machine created. In case you also have a machine there already you might want to just use that one. For the ones started from scratch I will show you now how to create a machine.
$ docker-machine create --driver virtualbox default
Running pre-create checks...
Creating machine...
(staging) Copying /Users/ripley/.docker/machine/cache/boot2docker.iso to /Users/ripley/.docker/machine/machines/default/boot2docker.iso...
(staging) Creating VirtualBox VM...
(staging) Creating SSH key...
(staging) Starting the VM...
(staging) Waiting for an IP...
Waiting for machine to be running, this may take a few minutes...
Machine is running, waiting for SSH to be available...
Detecting operating system of created instance...
Detecting the provisioner...
Provisioning with boot2docker...
Copying certs to the local machine directory...
Copying certs to the remote machine...
Setting Docker configuration on the remote daemon...
Checking connection to Docker...
Docker is up and running!
To see how to connect Docker to this machine, run: docker-machine env default
Note: In case you just created your machine from scratch, we first have to boot it. You can do so by running following command. In case your machine is already running you can skip this step.
docker-machine start
With the command shown previously we created a machine named default
using the virtualbox
driver. So when you run the docker-machine ls
command now, you should have similar output as you have seen before. You will also be able to see if the machine is running. We also see in the last line of the creation output that there is a special command which will provide you the information in order to connect your Docker client to this specific machine (docker-machine env default
). Lets configure the PowerShell environment to connect Docker to this specific machine.
$ docker-machine env default
$Env:DOCKER_TLS_VERIFY = "1"
$Env:DOCKER_HOST = "tcp://192.168.99.100:2376"
$Env:DOCKER_CERT_PATH = "C:\Users\marco\.docker\machine\machines\default"
$Env:DOCKER_MACHINE_NAME = "default"
# Run this command to configure your shell:
# & "C:\ProgramData\chocolatey\lib\docker-machine\bin\docker-machine.exe" env default | Invoke-Expression
Remember when you are running this command from Mac it will output it differently. The same goes when you are using Bash/Cygwin on Windows or when you are using the regular Command-Prompt on Windows. So just run the command in your preferred console and execute the command as shown in the output. In our case I'm going to continue using PowerShell, which means I will execute the command as we have just seen.
docker
Lets configure our PowerShell.
& "C:\ProgramData\chocolatey\lib\docker-machine\bin\docker-machine.exe" env default | Invoke-Expression
For Mac users the command will be something like following on bash.
eval $(docker-machine env default)
Now we will be able to use Docker on Windows. Lets try using the docker/whalesay image to test our Docker environment.
$ docker run docker/whalesay cowsay "Awesome your Docker environment is running!"
____________________________________
/ Awesome your Docker environment is \
\ running! /
------------------------------------
\
\
\
## .
## ## ## ==
## ## ## ## ===
/""""""""""""""""___/ ===
~~~ {~~ ~~~~ ~~~ ~~~~ ~~ ~ / ===- ~~~
\______ o __/
\ \ __/
\____\______/
In this article I won't go much deeper in the usage of Docker itself. So things like creating your own images, multi-container solutions and other more in depth Docker stuff won't be covered as it is out of scope. Therefore I only want to point you to docker --help
on your console and the Docker documentation for now, so I won't leave you completely in the dark. I might be publishing another post on how to run your project in Docker later.
Play time
The docker/whalesay
image also has command line help which reveals some more options (docker run docker/whalesay cowsay -h
). It reveals for example the whale can also think instead of talk (docker run docker/whalesay cowthink "Will he see the difference?"
). Have fun playing around with the docker/whalesay
image and the other options revealed in the help or simply try out some other images from the Docker hub.
Wrapup
If you finished playing around with Docker you might want to shutdown the VM using docker-machine stop default
. Next time you want to use it again simply run docker-machine start default
and docker-machine env default
to start and configure your environment. Last but not least I would like to point you to the --help
option. It will provide you with usage information on the command line options for every single command.
docker-machine --help
docker-machine create --help
TL;DR
As a bonus I want to give you all the commands we require to setup our environment in a PowerShell script. So feel free to save the contents in a ps1
file which you can call from your PowerShell.
Windows
cinst -y docker-machine
cinst -y docker
docker-machine create --driver virtualbox default
docker-machine start
& "C:\ProgramData\chocolatey\lib\docker-machine\bin\docker-machine.exe" env default | Invoke-Expression
docker run docker/whalesay cowsay -W 19 "Awesome your Docker environment is running!"
Mac
brew install docker-machine
brew install docker
docker-machine create --driver virtualbox default
docker-machine start
eval $(docker-machine env default)
docker run docker/whalesay cowsay -W 19 "Awesome your Docker environment is running!"
Finally, when you are finished playing arround with Docker, you might want to shut down your vm. You can do so by running.
docker-machine stop
In case you where one of the impatient ones, now realizing you have no clue what just happened when you called the commands in above example. Here you go for a second shot.
References:
Again thanks for reading this article, and as always share your feedback and questions in the comments.